Introduction to Basics a Software Engineer Should Know
Introduction
Hey, My name is Pavan and I am just any other software engineer with few years of experience so please excuse me if you find any mistakes in the series of posts that I am about to write. I hope you find these posts helpful and informative. I am always open to learning new things and improving my skills, so if you have any feedback or suggestions, please let me know.
Basics a Software Engineer Should Know
There is no substitute for the time and experience of working on the projects that teach you the most but there are some basics that every engineer should know. Here are some of the basics that I think every engineer should know to be effective. To be honest, there is a college degree with name compute science which is a good start but not all of us have that luxury or would have completely forgotten what we learned in college. Each of these topics is vast and requires a dedicated post to cover them in detail but I am not trying to repeat entire course work here but just trying to give a brief overview of the topics that I think are important. In the future, I will write dedicated posts on each of these topics to cover few additional details and provide the resources to explore further.
1. Computer Architecture & Memory Organization
Computer Architecture is the study of the design and organization of computer systems. It includes topics such as the design of the CPU, memory organization, input/output devices, and system buses. Computer architecture is an important topic for engineers to understand because it helps them understand how computers work and how they can write more efficient programs. Understanding computer architecture can help developers write programs that take advantage of the features of the hardware and improve the performance of their programs. It becomes very important if you are working on low level programming languages directly interacting with hardware but for high level programming languages, although Architecture is abstracted, it is still important to understand Memory Organization and how it works. So, let’s start with Memory Organization and we can cover Computer architecture topics as and when there is a need.
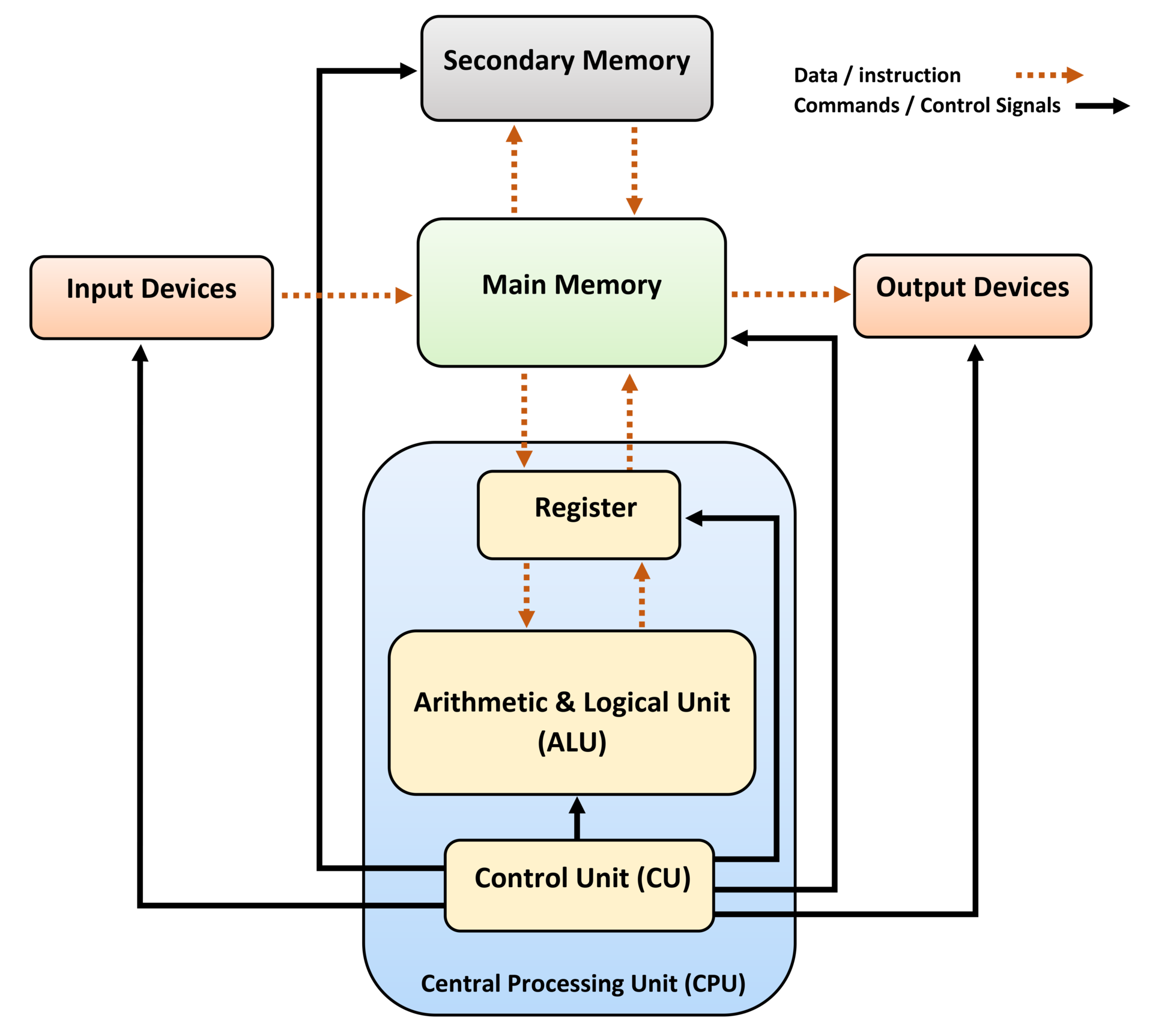
Credit: Computer Architecture Wiki
Memory is an essential part of a computer system. It is used to store data and instructions that are used by the CPU to perform operations. It can be divided into two types:
- Volatile Memory
- Non-Volatile Memory
Volatile memory is temporary and is lost when the power is turned off, while non-volatile memory is permanent and retains data even when the power is turned off. Volatile memory is further divided into two types
- Dynamic RAM (DRAM): Your typical RAM’s that you see in your computer.
- Static RAM (SRAM): Faster than DRAM and used in cache memory.
Non-volatile memory is divided into multiple types and important ones are:
- ROM (Read-Only Memory)
- Secondary Memory.
Your RAM and ROM are considered as primary/main memory and your hard disk, SSD, etc. are considered as secondary memory. Registers are the smallest and fastest type of memory and are used to store data and instructions that are currently being processed by the CPU and they are closer to the CPU than any other type of memory and provide the fastest access to data. Cache memory is used to store frequently accessed data and instructions to reduce the time it takes to access them. Main memory is used to store data and instructions that are currently being used by the CPU. Secondary memory is used to store data and instructions that are not currently being used by the CPU but need to be stored for future use. Memory is organized in a hierarchical manner. The memory hierarchy consists of different levels of memory, each with different characteristics. The memory hierarchy is organized in the following way:
- Registers
- Cache
- Main Memory
- Secondary Memory
Programs are loaded into memory from secondary memory and are executed by the CPU. The CPU fetches instructions and data from memory (registers, cache, main memory) and executes them. The memory hierarchy is used to provide the CPU with the data it needs in an efficient manner. The memory hierarchy is organized in such a way that the data that is most frequently accessed is stored in the fastest memory (registers, cache) and the data that is least frequently accessed is stored in the slowest memory (secondary memory). The memory hierarchy is used to reduce the time it takes to access data and instructions and improve the performance of the CPU. Data Structures and Algorithms essentially deal with space complexity and time complexity of the programs you have written and understanding memory organization can help you write more efficient data structures and algorithms. It is not necessary to understand memory organization to write programs but it can help you write more efficient programs. We will dive deep into memory organization in future posts.
I don’t want to overwhelm you with too much information in an introductory post and will stop here but in my head information is organized as a tree and I want to keep adding more branches to the tree. I am thinking of registers, program counter, memory address register, memory data register, etc about registers and how they work. CPU Cache Hierarchy, Memory Management Unit, Virtual Memory, etc as other topics to write but these topics do not rank high in my opinion from basics stand point. So for now all I want you to know is that memory is organized in a hierarchical manner and the memory hierarchy consists of different levels of memory, each with different characteristics.
If it peeked your curiousity you can read more about memory organization here.
2. Programming Languages & Data Structures and Algorithms
As the name suggest, Programming language is a language and is used by humans to communicate with computers. There are many programming languages with different syntax and semantics, but they all serve the same purpose: to write programs that tell the computer what to do. Programming languages are divided into two types:
- Low-Level Programming Languages
- High-Level Programming Languages
Low-level programming languages are closer to the hardware and are used to write programs that interact directly with the hardware. High-level programming languages are further divided into two types:
- Compiled Languages
- Interpreted Languages
Machine Code is the language that the computer understands and is used to execute programs. Assembly Language is a low-level programming language that is used to write programs that interact directly with the hardware. High-level programming languages are easier to write and understand than low-level programming languages and are used to write programs that are more complex and require more functionality. Compiled programming languages read the programs that you have written and convert them into machine code that the computer can understand. Interpreted programming languages read the programs that you have written and execute them line by line. There are pros and cons to each type and depends on the requirement of your project. Some Computer architecture understanding is required to be able to explain the difference of Low & High Level Programming Languages and Compiled & Interpreted Languages but it may be too much for this post. Essentially mastering one programming language is enough to get started in your engineering career but expanding your horizon to learn more programming languages will help you understand different techniques and approaches to solve problems.
Now with Programming language out of the way, Data Structures and Algorithms are next important topics. Essentially these topics are utilized in your program to store and manipulate data. Data structures are used to effectively organize data in memory so that the algorithms can be used to process the data stored in data structures and perform operations on it efficiently. Data structures and algorithms are used in almost every program that you write, so it is important to have a good understanding of them. It takes lot of time and practice but we can start with some basic data structures and algorithms and then move on to more complex ones. Some of the important data structures are:
- Arrays
- Linked Lists
- Stacks
- Queues
- Trees
- Graphs
- Hash Tables
- Heaps
Some important algorithms are:
- Sorting Algorithms
- Searching Algorithms
- Graph Algorithms
- Dynamic Programming
- Greedy Algorithms
- Divide and Conquer Algorithms
- Backtracking Algorithms
- Bit Manipulation
- Recursion
3. Operating Systems
Abstraction is core of Software Engineering and Operating System is the first abstraction that you encounter when you start writing programs. Essentially Operating System is a software that manages the hardware and provides interface/services to the user and other software. Operating System is an essential part of a computer system and is responsible for managing the resources of the computer, such as the CPU, memory, and input/output devices. There are many operating systems available today such as Windows, Linux, MacOS, being popular ones. I do not want to bring my opinion here but yet I would encourage you to start using Linux as it is open source and you can learn a lot about operating systems by using it. I am not clear in my head about the origins of Operating Systems but I think it started with batch processing systems and then moved to time sharing systems and then to multi-user systems. Initially BSD and Unix were popular but then Linux came into picture and now we have Windows, MacOS, etc. With different operating systems comes different file systems, different process management, different memory management, etc. POSIX is a standard that defines the API for Unix like operating systems and it is good to know about it. Many new techniques and processes are introduced in operating systems for each problem encountered and effectively many concepts of computer architecture, memory organization and programming languages are built into an operating system. Reviewing them will definitely help some of the problems we encounter in our programs. There are many concepts of operating systems that come in handy on a day to day basis such as
- Process Management
- Memory Management
- File System
- Device Management
Understanding these concepts will help you be more effective not only as a developer but also as a user of a computer system. We will dive deep into operating systems in future posts.
4. Computer Networks
Computer Networks are used to connect computers and other devices together so that they can communicate with each other. They are an essential part of a computer system and are used to transfer data between devices. There are many types of computer networks
- Local Area Network (LAN)
- Wide Area Network (WAN)
being popular ones. Concepts such as IP Address, Subnet Mask, Gateway, DNS, etc are important to understand how devices communicate with each other. Protocols such as TCP/IP, HTTP, FTP, etc are used to transfer data between devices. Understanding computer networks not only help you to write programs that communicate with other devices over a network but also help you to understand how the internet works. We will dive deep into computer networks in future posts.
5. Databases & Database Management Systems
Databases are used to store data and are an essential part of a computer system. You might be wondering there is already secondary memory to store data then why do we need databases. Many problems on data integrity, data consistency, data security, data redundancy, etc are solved by databases. Databases essentially provide a way to store data in an organized manner and retrieve it when needed and Database Management System is a software that is used to manage databases. There are many types of databases such as Relational Databases, NoSQL Databases, etc. SQL is a language that is used to interact with relational databases and is an essential skill for engineers to have. Understanding the volume of data, the speed at which data is accessed, the type of data that is stored, etc will help you choose the right database for your project and can improve the performance of your programs. Since databases are used widely in almost every program, conceptual understanding of databases is important. We will dive into
- ACID Properties
- Normalization
- Database Architecture
Conclusion
As you can imagine, there are many topics that are fundamental for Software Engineering and I have only scratched surface of Software Engineering. I am planning to touch on these topics and share interesting articles/videos as knowledge bytes. I hope you find these posts helpful and informative. I am always open to learning new things and improving my skills, so if you have any feedback or suggestions, please let me know. I am looking forward to hearing from you and I hope you enjoy reading these posts as much as I enjoy writing them. I will be writing more posts on these topics in the future and I hope you find them helpful. I am always open to feedback and suggestions, so please let me know if you have any. I am looking forward to hearing from you and I hope you enjoy reading these posts as much as I enjoy writing them. Thank you for reading and I hope you have a great day!
Version History:
- 1.0: Initial Post